Stripe Payment Gateway Integration in Laravel 9 with step-by-step Example
Hi Developers,
Today, we will be focusing on the Stripe Payment Gateway Integration in Laravel 9. Throughout this tutorial, we will guide you on how to integrate the Stripe payment gateway with Laravel 9. Additionally, you will gain hands-on experience with a practical example of Laravel 9 Stripe payment gateway integration. We will break down the steps involved in integrating Stripe payments with Laravel 9, providing you with a comprehensive understanding of the process.
To do Stripe Payment Gateway Integration in Laravel 9, you will first need to create a developer account on Stripe and obtain an API key and secret. Afterward, you can utilize the stripe/stripe-php composer library for the integration process. I will now provide a step-by-step guide on how to integrate the stripe payment gateway.
Stripe is a very popular and secure internet payment Merchant that helps to accept payments worldwide. Stripe provides really nice development interface to start and you don’t have to pay subscription charges to learn it provides a free developer account, before starting to code in your app.
I will give you an example from scratch to implement a stripe payment gateway in the laravel 9 application. You just need to follow a few steps to get a full example to pay.
Stripe Payment Gateway Integration in Laravel 9 with TutorialsOcean.com
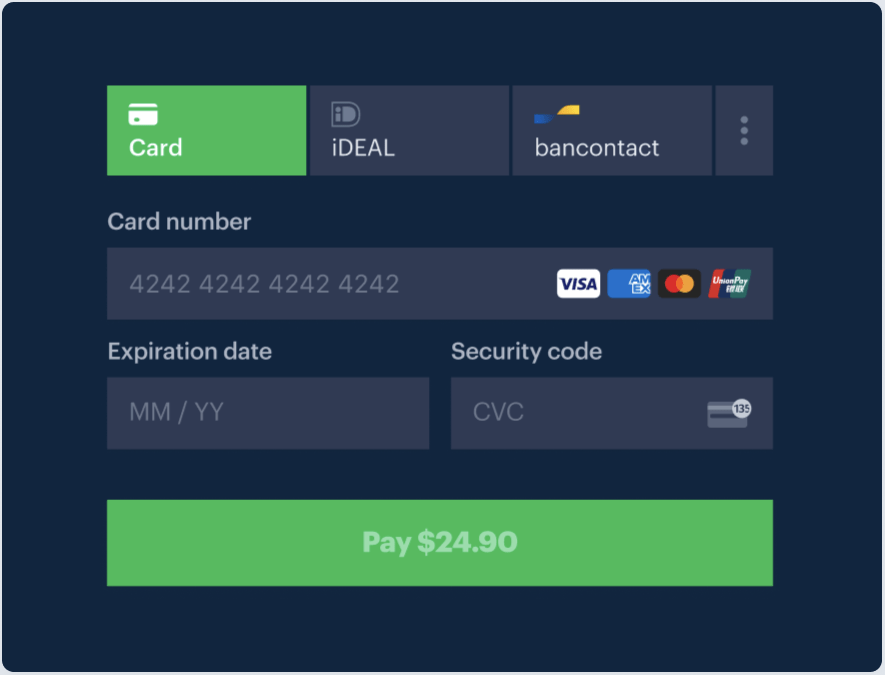
Step 1: Install Laravel App
In this step, You can create a Laravel 9 new project. So open the terminal and write the command.
composer create-project --prefer-dist laravel/laravel stripePayment
Step 2: Install stripe-php Package
In this step we need to install stripe-php via the Composer package manager, so one your terminal and fire the bellow command:
composer require stripe/stripe-php
Step 3: Set Stripe API Key and SECRET
Now, we need to set the stripe key and secret. so first you can go on the Stripe website and create a development stripe account key and secret and add bellow:
.env
STRIPE_KEY=pk_test_reFxwbsm9cdCKASdTfxAR
STRIPE_SECRET=sk_test_oQMFWteJiPd4wj4AtgApY
Step 4: Create Routes
In this step, we will create two routes for get request and another for post request. So, let’s add a new route to that file.
routes/web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\StripePaymentController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::get('stripe', [StripePaymentController::class, 'stripe']);
Route::post('stripe', [StripePaymentController::class, 'stripePost'])->name('stripe.post');
Step 5: Create Controller File
in the next step, we have to create a new controller as StripePaymentController and write both methods on it like as below, So let’s create the controller:
app/Http/Controllers/StripePaymentController.php
<?php
namespace App\Http\Controllers;
use Session;
use Stripe;
class StripePaymentController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function stripe()
{
return view('stripe');
}
public function stripe(Request $request)
{
Stripe\Stripe::setApiKey(env('STRIPE_SECRET'));
Stripe\Charge::create ([
"amount" => 100 * 100,
"currency" => "usd",
"source" => $request->stripeToken,
"description" => "Test payment from tutorialsocean.com."
]);
Session::flash('success', 'Payment successful!');
return back();
}
}
Step 6: Create Blade File
In the Last step, let’s create stripe.blade.php(resources/views/stripe.blade.php) for layout and write code of jquery to get token from stripe here and put the following code:
resources/views/stripe.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel 9 - Stripe Payment Gateway Integration Example - Tutorialsocean.com</title><link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<style type="text/css">
.panel-title {
display: inline;
font-weight: bold;
}
.display-table {
display: table;
}
.display-tr {
display: table-row;
}
.display-td {
display: table-cell;
vertical-align: middle;
width: 61%;
}
</style>
</head>
<body>
<div class="container">
<h1>Laravel 8 - Stripe Payment Gateway Integration Example <br/> ItSolutionStuff.com</h1>
<div class="row">
<div class="col-md-6 col-md-offset-3">
<div class="panel panel-default credit-card-box">
<div class="panel-heading display-table" >
<div class="row display-tr" >
<h3 class="panel-title display-td" >Payment Details</h3>
<div class="display-td" >
<img class="img-responsive pull-right" src="http://i76.imgup.net/accepted_c22e0.png">
</div>
</div>
</div>
<div class="panel-body">
@if (Session::has('success'))
<div class="alert alert-success text-center">
<a href="#" class="close" data-dismiss="alert" aria-label="close">×</a>
<p>{{ Session::get('success') }}</p>
</div>
@endif
<form
role="form"
action="{{ route('stripe.post') }}"
method="post"
class="require-validation"
data-cc-on-file="false"
data-stripe-publishable-key="{{ env('STRIPE_KEY') }}"
id="payment-form">
@csrf
<div class='form-row row'>
<div class='col-xs-12 form-group required'>
<label class='control-label'>Name on Card</label> <input
class='form-control' size='4' type='text'>
</div>
</div>
<div class='form-row row'>
<div class='col-xs-12 form-group card required'>
<label class='control-label'>Card Number</label> <input
autocomplete='off' class='form-control card-number' size='20'
type='text'>
</div>
</div>
<div class='form-row row'>
<div class='col-xs-12 col-md-4 form-group cvc required'>
<label class='control-label'>CVC</label> <input autocomplete='off'
class='form-control card-cvc' placeholder='ex. 311' size='4'
type='text'>
</div>
<div class='col-xs-12 col-md-4 form-group expiration required'>
<label class='control-label'>Expiration Month</label> <input
class='form-control card-expiry-month' placeholder='MM' size='2'
type='text'>
</div>
<div class='col-xs-12 col-md-4 form-group expiration required'>
<label class='control-label'>Expiration Year</label> <input
class='form-control card-expiry-year' placeholder='YYYY' size='4'
type='text'>
</div>
</div>
<div class='form-row row'>
<div class='col-md-12 error form-group hide'>
<div class='alert-danger alert'>Please correct the errors and try
again.</div>
</div>
</div>
<div class="row">
<div class="col-xs-12">
<button class="btn btn-primary btn-lg btn-block" type="submit">Pay Now ($100)</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</body>
<script type="text/javascript" src="https://js.stripe.com/v2/"></script>
<script type="text/javascript">
$(function() {
var $form = $(".require-validation");
$('form.require-validation').bind('submit', function(e) {
var $form = $(".require-validation"),
inputSelector = ['input[type=email]', 'input[type=password]',
'input[type=text]', 'input[type=file]',
'textarea'].join(', '),
$inputs = $form.find('.required').find(inputSelector),
$errorMessage = $form.find('div.error'),
valid = true;
$errorMessage.addClass('hide');
$('.has-error').removeClass('has-error');
$inputs.each(function(i, el) {
var $input = $(el);
if ($input.val() === '') {
$input.parent().addClass('has-error');
$errorMessage.removeClass('hide');
e.preventDefault();
}
});
if (!$form.data('cc-on-file')) {
e.preventDefault();
Stripe.setPublishableKey($form.data('stripe-publishable-key'));
Stripe.createToken({
number: $('.card-number').val(),
cvc: $('.card-cvc').val(),
exp_month: $('.card-expiry-month').val(),
exp_year: $('.card-expiry-year').val()
}, stripeResponseHandler);
}
});
function stripeResponseHandler(status, response) {
if (response.error) {
$('.error')
.removeClass('hide')
.find('.alert')
.text(response.error.message);
} else {
/* token contains id, last4, and card type */
var token = response['id'];
$form.find('input[type=text]').empty();
$form.append("<input type='hidden' name='stripeToken' value='" + token + "'/>");
$form.get(0).submit();
}
}
});
</script>
</html>
Now you can check with the following card details:
Name: Developer Number: 4242 4242 4242 4242 CSV: 123 Expiration Month: 12 Expiration Year: 2025